Learn How to Integrate Chat GPT Into Your Laravel 10 Application
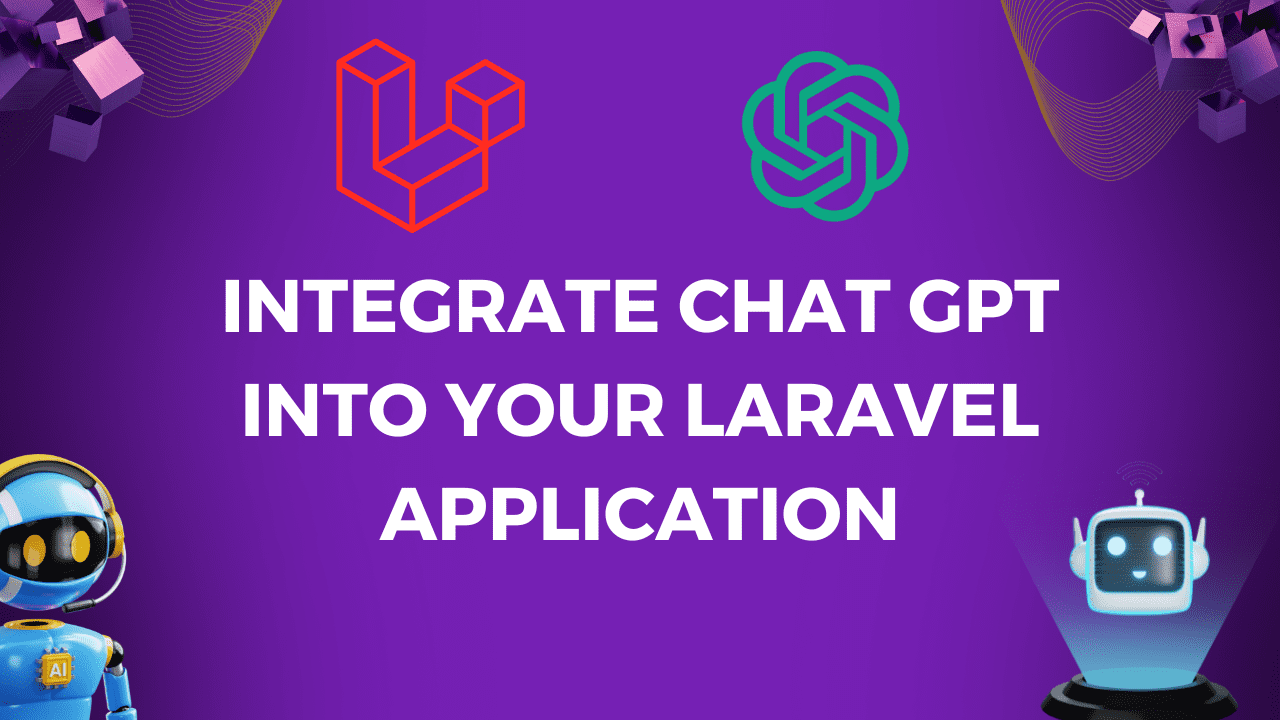
In today's date, Laravel is the best web framework, and with it, you can do almost anything. You can even integrate Chat GPT into it very easily; for this, you just have to follow some basic steps. So in this tutorial, we will tell you how you can integrate Chat GPT into your Laravel application very easily.
First of all, you have to install the latest Laravel project.
composer create-project laravel/laravel integrate-chat-gpt
If you have any existing projects, you can also use them.
After this, you have to open the environment file (.env) of your project. After opening the file, you have to copy the code given below and paste it in that file.
OPENAI_API="openai_api_key"
Here, you have to provide your API key. Make sure you replace this line "your_api_key" with Your API Key.
Now move on to the next step. In this step, you will have to install a package called openai-php/client. OpenAI PHP is a PHP API client for interacting with the Open AI API. To install it, you just have to run the command given below in your Laravel project.
composer require openai-php/client
Once this package is completely installed, you will have to create a service class file manually. Create this file by going to the app folder of your project, and in this folder you will also have to create a folder called Services. A file will be created inside this folder named as OpenAIService.php.
Then, in this newly created file, copy the code given below and paste it directly into that file.
<?php
namespace App\Services;
use OpenAI;
class OpenAIService
{
private $client;
public function __construct()
{
$this->client = OpenAI::client(env('OPENAI_API'));
}
public function generateResponseOpenAi(string $question): string
{
$response = $this->client->completions()->create([
'model' => 'text-davinci-003',
'temperature' => 0.9,
'top_p' => 1,
'frequency_penalty' => 0,
'presence_penalty' => 0,
'prompt' => $question,
'max_tokens' => 4000,
]);
return $response['choices'][0]['text'];
}
}
If you are making a chatbot and you want it to answer only those questions that you have already set in the backend, So you can use this given code instead of the above code. This code provides a way to generate responses using the OpenAI API based on a given question. If a question is found in the Qa model, it returns the corresponding answer; otherwise, it provides a default response.
<?php
namespace App\Services;
use OpenAI;
use App\Models\Qa;
class OpenAIService
{
private $client;
public function __construct()
{
$this->client = OpenAI::client(env('OPENAI_API'));
}
public function generateResponseOpenAi(string $question): string
{
$qa = Qa::where('question', $question)->first();
if ($qa) {
return $qa->answer;
}
$defaultResponse = "I'm sorry, I don't understand. Please ask a different question.";
return $defaultResponse;
}
}
Then you will need a controller because the controller receives a question from a request or a form, passes it to the OpenAIService to generate a response, and returns the response as a JSON object. Create the controller with this command.
php artisan make:controller ChatbotController
After that, you have to open that controller file and paste the below code in that file.
<?php
namespace App\Http\Controllers;
use App\Services\OpenAIService;
use Illuminate\Http\Request;
class ChatbotController extends Controller
{
private $openAiService;
public function __construct(OpenAIService $openAiService)
{
$this->openAiService = $openAiService;
}
public function chatOpenAi(Request $request)
{
$question = $request->question;
if ($question == null) {
return back();
}
$response= $this->openAiService->generateResponseOpenAi($question);
return response()->json(['response' => $response]);
}
}
All the work has been completed up to this point, now you just need to set up the routes and view file. Okay, first let's set up the routes. For this, you need to go inside the 'routes' folder of your project. There you will find a file named 'web.php'. Simply copy and paste the provided routes inside that file.
<?php
use App\Http\Controllers\ChatbotController;
// chat bot
Route::get('/chat-gpt-bot', function () {
return view('chat-bot');
})->name('chat-gpt-bot');
Route::match(['GET', 'POST'], '/chat-openai', [ChatbotController::class, 'chatOpenAi'])->name('chatOpenAi.send');
As we have defined a view in the first route, so we will create a file with the same name in the 'views' folder of our Laravel project. After creating the file, you just need to copy the provided code below and paste it into your file.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Learn How to Integrate Chat GPT Into Your Laravel 10 Application - ByteWebster</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css" rel="stylesheet">
<link rel="stylesheet" href="http://127.0.0.1:8000/main/plugins/fontawesome-free-6.4.0-web/css/all.min.css" />
<div class="container mt-4">
<div class="row">
<div class="col-md-6 offset-md-3">
<form id="chat-form" action="{{ route('chatOpenAi.send') }}" method="POST">
@csrf
<div class="input-group mb-3">
<input type="text" class="form-control" name="question" placeholder="Enter your question">
<div class="input-group-append">
<button class="btn btn-primary" type="submit">Ask</button>
</div>
</div>
</form>
<div class="card">
<div class="card-body">
<div id="response-container"></div>
</div>
</div>
</div>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/js/bootstrap.bundle.min.js"></script>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
$('#chat-form').submit(function(event) {
event.preventDefault();
var form = $(this);
var url = form.attr('action');
$.ajax({
type: 'POST',
url: url,
data: form.serialize(),
success: function(response) {
$('#response-container').append('<p>' + response.response + '</p>');
$('#chat-input').val('');
},
error: function(xhr, textStatus, errorThrown) {
console.log('Error:', errorThrown);
}
});
});
});
</script>
</body>
</html>
Up to this point, you have successfully integrated ChatGPT into Laravel, which is quite simple. Here, we have used Bootstrap 5 normally to create and design the view file. If you wish, you can also customize it according to your preferences.